使用 OpenCV 和 Python 在直播中模糊人脸
本文将学习如何使用 OpenCV 和 Python 在直播中模糊人脸。这将是一个非常有趣的博客,让我们开始吧!
我们最终结果的快照:
第 1 步:导入所需的库
· 为图像操作导入 cv2
· 为数组操作导入 Numpy
import cv2
import numpy as np
第 2 步:定义模糊函数
· 这里我们定义了 Blur 函数。
· 它需要 2 个参数,图像 img 和模糊因子 k 。
· 然后我们通过将高度和宽度除以模糊因子来简单地计算内核高度和内核宽度。kw 和 kh 越小,模糊度越高。
· 然后我们检查 kw 和 kh 是否为奇数,如果它们是偶数,则减 1 以使它们为奇数。
· 然后简单地我们将高斯模糊应用于我们的图像并返回它。
def blur(img,k):
h,w = img.shape[:2]
kh,kw = h//k,w//k
if kh%2==0:
kh-=1
if kw%2==0:
kw-=1
img = cv2.GaussianBlur(img,ksize=(kh,kw),sigmaX=0)
return img
第 3 步:定义 pixelate_face 函数
· 这是一个简单地为模糊图像添加像素化效果的函数。
def pixelate_face(image, blocks=10):
# divide the input image into NxN blocks
(h, w) = image.shape[:2]
xSteps = np.linspace(0, w, blocks + 1, dtype="int")
ySteps = np.linspace(0, h, blocks + 1, dtype="int")
# loop over the blocks in both the x and y direction
for i in range(1, len(ySteps)):
for j in range(1, len(xSteps)):
# compute the starting and ending (x, y)-coordinates
# for the current block
startX = xSteps[j - 1]
startY = ySteps[i - 1]
endX = xSteps[j]
endY = ySteps[i]
# extract the ROI using NumPy array slicing, compute the
# mean of the ROI, and then draw a rectangle with the
# mean RGB values over the ROI in the original image
roi = image[startY:endY, startX:endX]
(B, G, R) = [int(x) for x in cv2.mean(roi)[:3]]
cv2.rectangle(image, (startX, startY), (endX, endY),
(B, G, R), -1)
# return the pixelated blurred image
return image
第 4 步:让我们在实时提要中模糊面孔
· 下面的代码是代码的主要部分。
· 这里的 factor 定义了模糊量。
· 定义一个级联分类器对象 face_cascade 来检测人脸。
· 下载 haarcascade_frontalface_default.xml 文件
然后简单地运行一个无限循环,从网络摄像头读取图像,检测其中的人脸,然后用像素化的人脸替换该人脸部分。
阅读更多关于使用 HAARCASCADES 进行面部和眼睛检测的信息
factor = 3
cap = cv2.VideoCapture(0)
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
while 1:
ret,frame = cap.read()
gray = cv2.cvtColor(frame,cv2.COLOR_BGR2GRAY)
faces = face_cascade.detectMultiScale(gray, 1.5, 5)
for (x,y,w,h) in faces:
frame[y:y+h,x:x+w] = pixelate_face(blur(frame[y:y+h,x:x+w],factor))
cv2.imshow('Live',frame)
if cv2.waitKey(1)==27:
break
cap.release()
cv2.destroyAllWindows()
让我们看看完整代码
import cv2
import numpy as np
def blur(img,k):
h,w = img.shape[:2]
kh,kw = h//k,w//k
if kh%2==0:
kh-=1
if kw%2==0:
kw-=1
img = cv2.GaussianBlur(img,ksize=(kh,kw),sigmaX=0)
return img
def pixelate_face(image, blocks=10):
# divide the input image into NxN blocks
(h, w) = image.shape[:2]
xSteps = np.linspace(0, w, blocks + 1, dtype="int")
ySteps = np.linspace(0, h, blocks + 1, dtype="int")
# loop over the blocks in both the x and y direction
for i in range(1, len(ySteps)):
for j in range(1, len(xSteps)):
# compute the starting and ending (x, y)-coordinates
# for the current block
startX = xSteps[j - 1]
startY = ySteps[i - 1]
endX = xSteps[j]
endY = ySteps[i]
# extract the ROI using NumPy array slicing, compute the
# mean of the ROI, and then draw a rectangle with the
# mean RGB values over the ROI in the original image
roi = image[startY:endY, startX:endX]
(B, G, R) = [int(x) for x in cv2.mean(roi)[:3]]
cv2.rectangle(image, (startX, startY), (endX, endY),
(B, G, R), -1)
# return the pixelated blurred image
return image
factor = 3
cap = cv2.VideoCapture(0)
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
while 1:
ret,frame = cap.read()
gray = cv2.cvtColor(frame,cv2.COLOR_BGR2GRAY)
faces = face_cascade.detectMultiScale(gray, 1.5, 5)
for (x,y,w,h) in faces:
frame[y:y+h,x:x+w] = pixelate_face(blur(frame[y:y+h,x:x+w],factor))
cv2.imshow('Live',frame)
if cv2.waitKey(1)==27:
break
cap.release()
cv2.destroyAllWindows()
这就是你在直播中模糊面孔的方式!
原文标题 : 使用 OpenCV 和 Python 在直播中模糊人脸
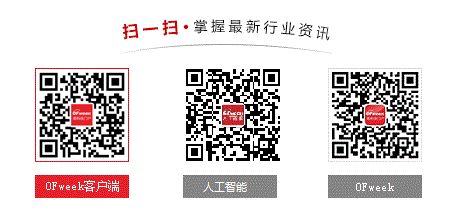
最新活动更多
-
3月27日立即报名>> 【工程师系列】汽车电子技术在线大会
-
4月1日立即下载>> 【村田汽车】汽车E/E架构革新中,新智能座舱挑战的解决方案
-
即日-4.22立即报名>> 【在线会议】汽车腐蚀及防护的多物理场仿真
-
4月23日立即报名>> 【在线会议】研华嵌入式核心优势,以Edge AI驱动机器视觉升级
-
4月25日立即报名>> 【线下论坛】新唐科技2025新品发布会
-
5月15日立即下载>> 【白皮书】精确和高效地表征3000V/20A功率器件应用指南
发表评论
请输入评论内容...
请输入评论/评论长度6~500个字
暂无评论
暂无评论